일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 | 31 |
- 막내의막무가내 rxjava
- 막내의막무가내 알고리즘
- 주엽역 생활맥주
- flutter network call
- 안드로이드 sunflower
- 막내의 막무가내 알고리즘
- 막내의 막무가내
- 안드로이드 Sunflower 스터디
- 막내의막무가내 플러터 flutter
- 막내의막무가내
- 막내의막무가내 코틀린
- 막내의막무가내 목표 및 회고
- 안드로이드
- 막내의막무가내 안드로이드 에러 해결
- 2022년 6월 일상
- Fragment
- 막내의막무가내 코틀린 안드로이드
- 프로그래머스 알고리즘
- 부스트코스
- 막내의막무가내 코볼 COBOL
- 막내의막무가내 플러터
- 막내의막무가내 프로그래밍
- 막내의막무가내 SQL
- 부스트코스에이스
- 주택가 잠실새내
- 프래그먼트
- 막내의막무가내 안드로이드 코틀린
- 막무가내
- 막내의막무가내 안드로이드
- 막내의막무가내 일상
- Today
- Total
막내의 막무가내 프로그래밍 & 일상
[알고리즘] 백준 15658 연산자 끼워넣기 (2) -백트래킹- 자바, 코틀린 본문
https://www.acmicpc.net/problem/15658
15658번: 연산자 끼워넣기 (2)
N개의 수로 이루어진 수열 A1, A2, ..., AN이 주어진다. 또, 수와 수 사이에 끼워넣을 수 있는 연산자가 주어진다. 연산자는 덧셈(+), 뺄셈(-), 곱셈(×), 나눗셈(÷)으로만 이루어져 있다. 연산자의 개수
www.acmicpc.net
백준 유형별풀기에 백트래킹 문제 연산자 끼워넣기 (2) 를 풀어봤습니다.
연산자 우선순위는 배제하고 정해지 연산자별 개수로 가장 큰 수식과 작은 수식 결과를 도출해내는 문제였습니다.
연산자 개수를 한개씩 줄여가며 백트래킹을 돌리면 되는 문제였습니다.
그리고 이렇게 두 개의 값을 연산하는 문제인 경우 첫번째 값을 넣어주고 돌리면 풀기 좋습니다 :)
풀이는 다음과 같습니다.
[Java]
import java.util.Scanner;
public class Main {
private static int N;
private static int[] op = new int[4];
private static int[] arr;
private static int max = Integer.MIN_VALUE;
private static int min = Integer.MAX_VALUE;
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
N = sc.nextInt();
arr = new int[N];
for (int i = 0; i < N; i++) {
arr[i] = sc.nextInt();
}
for (int i = 0; i < 4; i++) {
op[i] = sc.nextInt();
}
dfs(1, op, arr[0]);
System.out.println(max);
System.out.println(min);
}
private static void dfs(int depth, int[] op, int result) {
if (depth == N) {
max = Math.max(max, result);
min = Math.min(min, result);
return;
}
for (int i = 0; i < 4; i++) {
if (op[i] == 0) {
continue;
}
op[i]--;
switch (i) {
case 0:
dfs(depth + 1, op, result + arr[depth]);
break;
case 1:
dfs(depth + 1, op, result - arr[depth]);
break;
case 2:
dfs(depth + 1, op, result * arr[depth]);
break;
case 3:
dfs(depth + 1, op, result / arr[depth]);
break;
}
op[i]++;
}
}
}
[Kotlin]
import java.util.*
private var N = 0
private val op = IntArray(4)
private lateinit var arr: IntArray
private var max = Int.MIN_VALUE
private var min = Int.MAX_VALUE
fun main(args: Array<String>) {
val sc = Scanner(System.`in`)
N = sc.nextInt()
arr = IntArray(N)
for (i in 0 until N) {
arr[i] = sc.nextInt()
}
for (i in 0..3) {
op[i] = sc.nextInt()
}
dfs(1, op, arr[0])
println(max)
println(min)
}
private fun dfs(depth: Int, op: IntArray, result: Int) {
if (depth == N) {
max = Math.max(max, result)
min = Math.min(min, result)
return
}
for (i in 0..3) {
if (op[i] == 0) {
continue
}
op[i]--
when (i) {
0 -> dfs(depth + 1, op, result + arr[depth])
1 -> dfs(depth + 1, op, result - arr[depth])
2 -> dfs(depth + 1, op, result * arr[depth])
3 -> dfs(depth + 1, op, result / arr[depth])
}
op[i]++
}
}
https://github.com/mtjin/algorithm_practice/commit/d982e5e058e2b74ea434ce44efb8fd68bf92ea4f
백준 15658 풀이 · mtjin/algorithm_practice@d982e5e
Permalink This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository. Showing 2 changed files with 96 additions and 0 deletions. +53 −0 백준 15658 연산자 끼워넣기 (2) -백트래킹-/Main.java
github.com
오랜만에 자바와 코틀린으로 알고리즘을 풀어봤네요.
뇌가 굳지 않게 꾸준히 해야겠습니다...
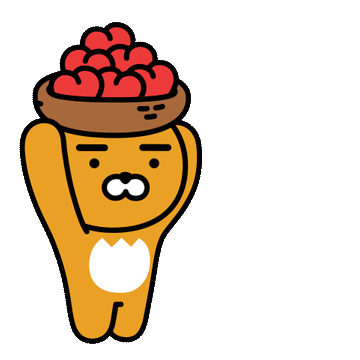
댓글과 공감은 큰 힘이 됩니다. 감사합니다. !!!
'알고리즘 > DFS, BFS, 시뮬, 백트래킹' 카테고리의 다른 글
[알고리즘] 백준 16922 로마 숫자 만들기 -백트래킹- 자바, 코틀린 (0) | 2022.04.30 |
---|---|
[알고리즘] 백준 10974 모든 순열 -백트래킹- 자바, 코틀린 (2) | 2022.01.04 |
[알고리즘] 백준 15656 N과 M (7) -백트래킹- 자바 (2) | 2021.11.22 |
[알고리즘] 프로그래머스 가장 큰 정사각형 찾기 -BFS, DP- 자바 (0) | 2021.08.10 |
[알고리즘] 백준 1405 미친 로봇 -DFS, 완전탐색- 자바 코틀린 (0) | 2021.08.09 |