일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | |
7 | 8 | 9 | 10 | 11 | 12 | 13 |
14 | 15 | 16 | 17 | 18 | 19 | 20 |
21 | 22 | 23 | 24 | 25 | 26 | 27 |
28 | 29 | 30 |
- Fragment
- 막내의막무가내 SQL
- 막내의막무가내 안드로이드 에러 해결
- 막내의막무가내 안드로이드
- 막내의막무가내 프로그래밍
- 프래그먼트
- 막내의막무가내
- 안드로이드
- 막내의막무가내 코틀린
- 부스트코스
- 막내의막무가내 목표 및 회고
- 막내의막무가내 rxjava
- 막내의막무가내 안드로이드 코틀린
- 주엽역 생활맥주
- flutter network call
- 막내의막무가내 코틀린 안드로이드
- 막내의막무가내 플러터 flutter
- 막내의막무가내 일상
- 막무가내
- 부스트코스에이스
- 2022년 6월 일상
- 막내의막무가내 플러터
- 막내의 막무가내 알고리즘
- 주택가 잠실새내
- 막내의막무가내 코볼 COBOL
- 프로그래머스 알고리즘
- 안드로이드 Sunflower 스터디
- 안드로이드 sunflower
- 막내의 막무가내
- 막내의막무가내 알고리즘
- Today
- Total
막내의 막무가내 프로그래밍 & 일상
[Flutter] Udemy 플러터 강의 섹션 3 학습 (I Am Rich - How to Create Flutter Apps FromScratch) 본문
[Flutter] Udemy 플러터 강의 섹션 3 학습 (I Am Rich - How to Create Flutter Apps FromScratch)
막무가내막내 2021. 8. 14. 23:14
https://www.udemy.com/course/flutter-bootcamp-with-dart/
The Complete 2021 Flutter Development Bootcamp with Dart
Officially created in collaboration with the Google Flutter team.
www.udemy.com
안젤라님의 Udemy 강의를 구입하여 플러터 기본기를 공부하고 있습니다.
섹션 2까지는 플러터란 무엇인가에 대해 배울 수 있었고 기본적인 플러터 세팅을 마쳤습니다.
https://github.com/mtjin/flutter-practice
GitHub - mtjin/flutter-practice: Learning About Flutter (플러터 공부)
Learning About Flutter (플러터 공부). Contribute to mtjin/flutter-practice development by creating an account on GitHub.
github.com
섹션 3부터는 이제 코드 실습과 함께 기본기를 배워나가는 것 같습니다.
이 강의에서 클론 코딩하며 배운 것들은 위 깃허브 레포지토리에 기록할 예정입니다.
추가로 섹션별로 나눠 배운 것이나 코드들을 나중에 복습 및 참고를 위해 정리하려고 합니다.
설명은 주석 위주로 남기겠습니다.
[24. Creating a New Flutter Project from Scratch]
import 'package:flutter/material.dart';
// main() 은 모든 플러터앱의 시작점이다.
void main() {
runApp(MaterialApp(
home: Center(
child: Text("Hello World"),
),
));
}
[26. Scaffolding a Flutter App]
참고할 공식문서
https://api.flutter.dev/flutter/material/Scaffold-class.html
Scaffold class - material library - Dart API
Implements the basic material design visual layout structure. This class provides APIs for showing drawers and bottom sheets. To display a persistent bottom sheet, obtain the ScaffoldState for the current BuildContext via Scaffold.of and use the ScaffoldSt
api.flutter.dev
Scaffold란?
기본 머터리얼 디자인 레이아웃 구조를 구현해놓은거라고 보면 됩니다.
또한 레이아웃 구조를 머터리얼 디자인으로 쉽게 만들 수 있도록 여러가지 속성들을 가지고 있습니다.
추가적인 팁으로 윈도우 기준 Alt + Enter 로 속성들을 쉽게 추가할 수 있습니다.
import 'package:flutter/material.dart';
// main() 은 모든 플러터앱의 시작점이다.
void main() {
runApp(MaterialApp(
home: Scaffold(
backgroundColor: Colors.blueGrey,
appBar: AppBar(
title: Text("I Am Rich"),
backgroundColor: Colors.blueGrey[900],
),
body: Center(
child: Image( // NetworkImage는 Url을 통해 서버이미지를 가져올때 사용합니다. 안드로이드의 Glide라고 생각!, 그밖에 AssetImage 등 다른것들도 있습니다.
image: NetworkImage('https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcQnYB7pV4de12zrKQayds0fU56TBYw06fYaqQ&usqp=CAU'),
),
),
)
));
}
Scaffold 구조로 그림을 레이아웃 구조를 그려봤고 이를 구조도로 나타내면 다음과 같을 것 입니다.
[27. Working with Assets in Flutter & the Pubspec file]
이전 강의에서는 url로 이미지를 불러왔다면 이번에는 프로젝트 내부 이미지 파일을 불러오는 방법에 대해 알아봤습니다.
먼저 images 라는 디렉토리를 만들어주고 강의에서 제공한 이미지를 해당 디렉토리에 넣어줍니다.
그 후 assets 을 애플리케이션에 추가해주기 위해 pubspec.yml에서 assets: 라고 주석이 되어있는 부분으로 갑니다.
yml파일은 configuration(구성, 환경) 을 설정해주는 파일이라고 생각하며 편합니다.
이 주석된 부분에 제가 추가한 이미지 파일을 추가(명시)해주도록 합니다. 이렇게함으로써 제 프로젝트의 dart파일에서 이미지를 불러올 수 있도록 합니다.
그리고 yaml파일에서 주의할 점은 파이썬처럼 인덴트(indentation)에 조심해야합니다. 투 Space 규칙을 지켜야하고 안지킬시 에러가 납니다!
그리고 위처럼 이미지파일을 써도 되지만 그러면 이미지 파일을 한개 추가할때마다 추가로 적어줘야하므로 매우 비효율적일 것입니다. 이를 위와 같이 루트 디렉토리만 작성하면 해결됩니다.
마지막으로 우측상단의 Pub get을 클릭해주면 됩니다.
import 'package:flutter/material.dart';
// main() 은 모든 플러터앱의 시작점이다.
void main() {
runApp(MaterialApp(
home: Scaffold(
backgroundColor: Colors.blueGrey,
appBar: AppBar(
title: Text("I Am Rich"),
backgroundColor: Colors.blueGrey[900],
),
body: Center(
child: Image( // AssetImage 는 프로젝트 내부 이미지 파일을 불러올 수 있습니다.
image: AssetImage('images/diamond.png'),
),
),
)
));
}
AssetImage() 로 이제 프로젝트 내부 이미지 파일을 불러올 수 있고 결과는 다음과 같습니다.
[28. How to Add App Icons to the iOS and Android Apps]
이미지를 IOS, ANDROID 앱 아이콘을 만들어주는 사이트입니다.
App Icon Generator
appicon.co
이미지를 추가해주고 Generate 해주고 파일을 다운받으면 됩니다.
이제 앱 아이콘을 Android와 IOS에 추가해야하는데 디렉토리 구조를 보시면 Android와 IOS가 디렉토리가 보일겁니다.
먼저 안드로이드에 앱 아이콘을 추가하려면 위와 같이 mipmap 디렉토리에 제가 앞서 다운받은 아이콘이미지 파일들을 추가해주면 됩니다. 쉽게 한번에 삭제하고 추가하려면 해당 mipmap 디렉토리 마우스 우클릭 후 Show in Explorer 해서 mipmap 디렉토리들을 모두 삭제하고 제가 다운 받은 mipmap 폴더들을 추가해주면 됩니다.
추가로 이러한 방식말고도 기존의 안드로이드 스튜디오에서 앱 아이콘 Asset파일을 만들듯이 추가할수도 있습니다.
IOS의 경우는 위 디렉토리의 이미지를 변경해주면 됩니다.
앱 아이콘이 적용된 모습을 볼 수 있습니다 :)
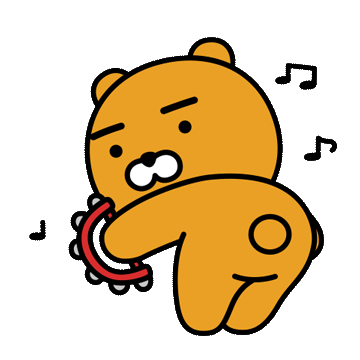
이상 섹션3 강의를 모두 마쳤습니다.
댓글과 공감은 큰 힘이 됩니다. 감사합니다.
[다음 학습]
https://youngest-programming.tistory.com/606
[Flutter] Udemy 플러터 강의 섹션 4 학습 (학습예정)
[이전학습] https://youngest-programming.tistory.com/604 [Flutter] Udemy 플러터 강의 섹션 3 학습 (학습중) https://www.udemy.com/course/flutter-bootcamp-with-dart/learn/lecture/14481952#overview 안젤..
youngest-programming.tistory.com
'플러터(Flutter) & Dart' 카테고리의 다른 글
[플러터] Flutter 참고하면 좋은 사이트 모음 (0) | 2021.08.24 |
---|---|
[Flutter] Udemy 플러터 강의 섹션 6 학습 (MiCard - How to Build Beautiful UIs with FlutterWidgets) (0) | 2021.08.19 |
[Flutter] Udemy 플러터 강의 섹션 5 학습 (I Am Poor - App Challenge) (4) | 2021.08.17 |
[Flutter] Udemy 플러터 강의 섹션 4 학습 (Running Your App on a Physical Device) (0) | 2021.08.17 |
[Flutter] 플러터 공부 및 정리 (공부 예정) (2) | 2021.01.15 |