일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 | 31 |
- 막내의막무가내 코틀린
- 안드로이드
- 막내의막무가내 코볼 COBOL
- 2022년 6월 일상
- flutter network call
- 막내의막무가내 rxjava
- 안드로이드 Sunflower 스터디
- 막내의막무가내
- 막내의막무가내 프로그래밍
- Fragment
- 프래그먼트
- 막내의막무가내 목표 및 회고
- 막내의막무가내 SQL
- 막내의 막무가내
- 막내의 막무가내 알고리즘
- 부스트코스
- 부스트코스에이스
- 막내의막무가내 안드로이드 에러 해결
- 막내의막무가내 안드로이드 코틀린
- 막내의막무가내 안드로이드
- 안드로이드 sunflower
- 막내의막무가내 플러터
- 주엽역 생활맥주
- 막내의막무가내 알고리즘
- 막무가내
- 막내의막무가내 코틀린 안드로이드
- 막내의막무가내 플러터 flutter
- 프로그래머스 알고리즘
- 주택가 잠실새내
- 막내의막무가내 일상
- Today
- Total
막내의 막무가내 프로그래밍 & 일상
[Flutter] Udemy 플러터 강의 섹션 10 학습 (섹션 10: Quizzler -Modularising & Organising FlutterCode) 본문
[Flutter] Udemy 플러터 강의 섹션 10 학습 (섹션 10: Quizzler -Modularising & Organising FlutterCode)
막무가내막내 2021. 9. 26. 11:20
[이전학습]
https://youngest-programming.tistory.com/623
[Flutter] Udemy 플러터 강의 섹션 9 학습 (Xylophone - Using Flutter and Dart Packages toSpeed Up Development)
[이전학습] https://youngest-programming.tistory.com/621 [Flutter] Udemy 플러터 강의 섹션 8 학습 (학습중..) (Boss Level Challenge 1 - Magic 8 Ball) [이전학습] https://youngest-programming.tistory.co..
youngest-programming.tistory.com
[참고]
https://www.udemy.com/course/flutter-bootcamp-with-dart/
The Complete 2021 Flutter Development Bootcamp with Dart
Officially created in collaboration with the Google Flutter team.
www.udemy.com
https://github.com/mtjin/flutter-practice
GitHub - mtjin/flutter-practice: Learning About Flutter (플러터 공부)
Learning About Flutter (플러터 공부). Contribute to mtjin/flutter-practice development by creating an account on GitHub.
github.com
[87. Quizzler - A True/False Quiz App]
정보처리기사 공부할때 퀴즈렛을 한적이 있는데 그거와 비슷한 Quizzler 라는 앱을 만들어볼 예정입니다.
[88. Building a Score Keeper]
리스트 사용법과 리스트를 활용하여 True 버튼 클릭 시 동적으로 아이콘을 추가하는 방법에 대해 배웠습니다.
import 'package:flutter/material.dart';
void main() => runApp(Quizzler());
class Quizzler extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: Colors.grey.shade900,
body: SafeArea(
child: Padding(
padding: EdgeInsets.symmetric(horizontal: 10.0),
child: QuizPage(),
),
),
),
);
}
}
class QuizPage extends StatefulWidget {
@override
_QuizPageState createState() => _QuizPageState();
}
class _QuizPageState extends State<QuizPage> {
// 스코어 아이콘 리스트
List<Icon> scoreKeeper = [
Icon(
Icons.check,
color: Colors.green,
),
Icon(
Icons.close,
color: Colors.red,
),
Icon(
Icons.close,
color: Colors.red,
),
Icon(
Icons.close,
color: Colors.red,
),
Icon(
Icons.close,
color: Colors.red,
),
];
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Expanded(
flex: 5,
child: Padding(
padding: EdgeInsets.all(10.0),
child: Center(
child: Text(
'This is where the question text will go.',
textAlign: TextAlign.center,
style: TextStyle(
fontSize: 25.0,
color: Colors.white,
),
),
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
textColor: Colors.white,
color: Colors.green,
child: Text(
'True',
style: TextStyle(
color: Colors.white,
fontSize: 20.0,
),
),
onPressed: () {
//The user picked true.
setState(() {
scoreKeeper.add(Icon(
Icons.check,
color: Colors.green,
));
});
},
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
color: Colors.red,
child: Text(
'False',
style: TextStyle(
fontSize: 20.0,
color: Colors.white,
),
),
onPressed: () {
//The user picked false.
},
),
),
),
// 스코어 리스트 동적으로 그려줌
Row(children: scoreKeeper)
],
);
}
}
/*
question1: 'You can lead a cow down stairs but not up stairs.', false,
question2: 'Approximately one quarter of human bones are in the feet.', true,
question3: 'A slug\'s blood is green.', true,
*/
[89. [Dart] Lists]
다트의 리스트 개념에 대해 배웠습니다.
자바와 리스트 선언 및 함수 활용법이 크게 다른점이 없었습니다.
[90. Displaying the Questions]
질문 리스트와 질문 번호 변수를 만들어주었습니다.
그리고 true, false 클릭 시 질문번호가 증가되게 하고 질문번호가 변경되게 텍스트를 세팅해주었습니다.
주의할 점은 다음과 같습니다.
// 질문 번호 (핫리로드시 state를 보존하기때문에 이 값을 보존함 0부터 다시 시작하고싶으면 핫스타트 사용해야함)
int questionNumber = 0;
import 'package:flutter/material.dart';
void main() => runApp(Quizzler());
class Quizzler extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: Colors.grey.shade900,
body: SafeArea(
child: Padding(
padding: EdgeInsets.symmetric(horizontal: 10.0),
child: QuizPage(),
),
),
),
);
}
}
class QuizPage extends StatefulWidget {
@override
_QuizPageState createState() => _QuizPageState();
}
class _QuizPageState extends State<QuizPage> {
// 스코어 아이콘 리스트
List<Icon> scoreKeeper = [];
// 질문리스트
List<String> questions = [
'You can lead a cow down stairs but not up stairs.',
'Approximately one quarter of human bones are in the feet.',
'A slug\'s blood is green.'
];
// 질문 번호 (핫리로드시 state를 보존하기때문에 이 값을 보존함 0부터 다시 시작하고싶으면 핫스타트 사용해야함)
int questionNumber = 0;
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Expanded(
flex: 5,
child: Padding(
padding: EdgeInsets.all(10.0),
child: Center(
child: Text(
questions[questionNumber], //리스트 이용
textAlign: TextAlign.center,
style: TextStyle(
fontSize: 25.0,
color: Colors.white,
),
),
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
textColor: Colors.white,
color: Colors.green,
child: Text(
'True',
style: TextStyle(
color: Colors.white,
fontSize: 20.0,
),
),
onPressed: () {
//The user picked true.
setState(() {
questionNumber++;
});
},
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
color: Colors.red,
child: Text(
'False',
style: TextStyle(
fontSize: 20.0,
color: Colors.white,
),
),
onPressed: () {
//The user picked false.
setState(() {
questionNumber++;
});
},
),
),
),
// 스코어 리스트 동적으로 그려줌
Row(children: scoreKeeper)
],
);
}
}
/*
question1: 'You can lead a cow down stairs but not up stairs.', false,
question2: 'Approximately one quarter of human bones are in the feet.', true,
question3: 'A slug\'s blood is green.', true,
*/
[91. Checking User Answers]
정답 리스트를 추가 하고
if else 문 활용법에 대해 배웠습니다.
import 'package:flutter/material.dart';
void main() => runApp(Quizzler());
class Quizzler extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: Colors.grey.shade900,
body: SafeArea(
child: Padding(
padding: EdgeInsets.symmetric(horizontal: 10.0),
child: QuizPage(),
),
),
),
);
}
}
class QuizPage extends StatefulWidget {
@override
_QuizPageState createState() => _QuizPageState();
}
class _QuizPageState extends State<QuizPage> {
// 스코어 아이콘 리스트
List<Icon> scoreKeeper = [];
// 질문리스트
List<String> questions = [
'You can lead a cow down stairs but not up stairs.',
'Approximately one quarter of human bones are in the feet.',
'A slug\'s blood is green.'
];
// 정답리스트
List<bool> answers = [false, true, true];
// 질문 번호 (핫리로드시 state를 보존하기때문에 이 값을 보존함 0부터 다시 시작하고싶으면 핫스타트 사용해야함)
int questionNumber = 0;
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Expanded(
flex: 5,
child: Padding(
padding: EdgeInsets.all(10.0),
child: Center(
child: Text(
questions[questionNumber], //리스트 이용
textAlign: TextAlign.center,
style: TextStyle(
fontSize: 25.0,
color: Colors.white,
),
),
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
textColor: Colors.white,
color: Colors.green,
child: Text(
'True',
style: TextStyle(
color: Colors.white,
fontSize: 20.0,
),
),
onPressed: () {
//The user picked true.
bool correctNumber = answers[questionNumber];
// if else 문을 활용한 정답 분기처리
if (correctNumber == true) {
print('right');
} else {
print('wrong');
}
setState(() {
questionNumber++;
});
},
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
color: Colors.red,
child: Text(
'False',
style: TextStyle(
fontSize: 20.0,
color: Colors.white,
),
),
onPressed: () {
//The user picked false.
setState(() {
questionNumber++;
});
},
),
),
),
// 스코어 리스트 동적으로 그려줌
Row(children: scoreKeeper)
],
);
}
}
/*
question1: 'You can lead a cow down stairs but not up stairs.', false,
question2: 'Approximately one quarter of human bones are in the feet.', true,
question3: 'A slug\'s blood is green.', true,
*/
[92. [Dart] Conditionals - IF/ELSE]
Dart의 if else 문 개념과 사용법에 대해 배웠는데 이전 언어와 동일하였습니다.
[94. Creating a Question Class]
기존에 문제이름(String)과 정답(bool)로 이루어진 구조를 클래스를 활용하여 한번에 묶어줌으로써 더 효율적으로 코드를 변경하였습니다.
처음 다트 클래스를 활용해보았는데 기존 자바와 크게 다른점은 없었습니다.
class Question {
String questionText;
bool questionAnswer;
Question({String q, bool a}) {
questionText = q;
questionAnswer = a;
}
}
import 'package:flutter/material.dart';
import 'question.dart';
void main() => runApp(Quizzler());
class Quizzler extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: Colors.grey.shade900,
body: SafeArea(
child: Padding(
padding: EdgeInsets.symmetric(horizontal: 10.0),
child: QuizPage(),
),
),
),
);
}
}
class QuizPage extends StatefulWidget {
@override
_QuizPageState createState() => _QuizPageState();
}
class _QuizPageState extends State<QuizPage> {
// 스코어 아이콘 리스트
List<Icon> scoreKeeper = [];
// 클래스 리스트
List<Question> questionBank = [
Question(q: 'You can lead a cow down stairs but not up stairs.', a: false),
Question(
q: 'Approximately one quarter of human bones are in the feet.',
a: true),
Question(q: 'A slug\'s blood is green.', a: true),
];
// 질문 번호 (핫리로드시 state를 보존하기때문에 이 값을 보존함 0부터 다시 시작하고싶으면 핫스타트 사용해야함)
int questionNumber = 0;
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Expanded(
flex: 5,
child: Padding(
padding: EdgeInsets.all(10.0),
child: Center(
child: Text(
questionBank[questionNumber].questionText, //리스트 이용
textAlign: TextAlign.center,
style: TextStyle(
fontSize: 25.0,
color: Colors.white,
),
),
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
textColor: Colors.white,
color: Colors.green,
child: Text(
'True',
style: TextStyle(
color: Colors.white,
fontSize: 20.0,
),
),
onPressed: () {
//The user picked true.
bool correctNumber =
questionBank[questionNumber].questionAnswer;
// if else 문을 활용한 정답 분기처리
if (correctNumber == true) {
print('right');
} else {
print('wrong');
}
setState(() {
questionNumber++;
});
},
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
color: Colors.red,
child: Text(
'False',
style: TextStyle(
fontSize: 20.0,
color: Colors.white,
),
),
onPressed: () {
//The user picked false.
setState(() {
questionNumber++;
});
},
),
),
),
// 스코어 리스트 동적으로 그려줌
Row(children: scoreKeeper)
],
);
}
}
/*
question1: 'You can lead a cow down stairs but not up stairs.', false,
question2: 'Approximately one quarter of human bones are in the feet.', true,
question3: 'A slug\'s blood is green.', true,
*/
[95. [Dart] Classes and Objects]
다트의 클래스와 객체 개념과 활용법에 대해 배웠습니다. 기존에 알고 있던 언어와 큰 차이점은 없고 동일했습니다.
[96. Abstraction in Action]
QuizBrain 이라는 Object 클래스를 만들어주어 Question 리스트를 관리해주는 식으로 코드를 변경했습니다. 모듈화를 한 것이라 보면 됩니다.
import 'question.dart';
class QuizBrain {
List<Question> questionBank = [
Question('Some cats are actually allergic to humans', true),
Question('You can lead a cow down stairs but not up stairs.', false),
Question('Approximately one quarter of human bones are in the feet.', true),
Question('A slug\'s blood is green.', true),
Question('Buzz Aldrin\'s mother\'s maiden name was \"Moon\".', true),
Question('It is illegal to pee in the Ocean in Portugal.', true),
Question(
'No piece of square dry paper can be folded in half more than 7 times.',
false),
Question(
'In London, UK, if you happen to die in the House of Parliament, you are technically entitled to a state funeral, because the building is considered too sacred a place.',
true),
Question(
'The loudest sound produced by any animal is 188 decibels. That animal is the African Elephant.',
false),
Question(
'The total surface area of two human lungs is approximately 70 square metres.',
true),
Question('Google was originally called \"Backrub\".', true),
Question(
'Chocolate affects a dog\'s heart and nervous system; a few ounces are enough to kill a small dog.',
true),
Question(
'In West Virginia, USA, if you accidentally hit an animal with your car, you are free to take it home to eat.',
true),
];
}
import 'package:flutter/material.dart';
import 'package:quizzler/quiz_brain.dart';
QuizBrain quizBrain = QuizBrain();
void main() => runApp(Quizzler());
class Quizzler extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: Colors.grey.shade900,
body: SafeArea(
child: Padding(
padding: EdgeInsets.symmetric(horizontal: 10.0),
child: QuizPage(),
),
),
),
);
}
}
class QuizPage extends StatefulWidget {
@override
_QuizPageState createState() => _QuizPageState();
}
class _QuizPageState extends State<QuizPage> {
// 스코어 아이콘 리스트
List<Icon> scoreKeeper = [];
// 질문 번호 (핫리로드시 state를 보존하기때문에 이 값을 보존함 0부터 다시 시작하고싶으면 핫스타트 사용해야함)
int questionNumber = 0;
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Expanded(
flex: 5,
child: Padding(
padding: EdgeInsets.all(10.0),
child: Center(
child: Text(
quizBrain.questionBank[questionNumber].questionText, //리스트 이용
textAlign: TextAlign.center,
style: TextStyle(
fontSize: 25.0,
color: Colors.white,
),
),
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
textColor: Colors.white,
color: Colors.green,
child: Text(
'True',
style: TextStyle(
color: Colors.white,
fontSize: 20.0,
),
),
onPressed: () {
//The user picked true.
bool correctNumber =
quizBrain.questionBank[questionNumber].questionAnswer;
// if else 문을 활용한 정답 분기처리
if (correctNumber == true) {
print('right');
} else {
print('wrong');
}
setState(() {
questionNumber++;
});
},
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
color: Colors.red,
child: Text(
'False',
style: TextStyle(
fontSize: 20.0,
color: Colors.white,
),
),
onPressed: () {
//The user picked false.
setState(() {
questionNumber++;
});
},
),
),
),
// 스코어 리스트 동적으로 그려줌
Row(children: scoreKeeper)
],
);
}
}
/*
question1: 'You can lead a cow down stairs but not up stairs.', false,
question2: 'Approximately one quarter of human bones are in the feet.', true,
question3: 'A slug\'s blood is green.', true,
*/
[97. Encapsulation in Action]
QuizBrain에 변수와 함수를 추가함으로써 더욱 모듈화 시켰습니다.
또한 캡슐화를 시켜서 get은 가능하나 set은 못하게함으로써 불변하는것들에 대한 수정하는 실수가 없도록 구현했습니다.
import 'question.dart';
class QuizBrain {
// 질문 번호 (핫리로드시 state를 보존하기때문에 이 값을 보존함 0부터 다시 시작하고싶으면 핫스타트 사용해야함)
int _questionNumber = 0;
// 캡슐화시킴
List<Question> _questionBank = [
Question('Some cats are actually allergic to humans', true),
Question('You can lead a cow down stairs but not up stairs.', false),
Question('Approximately one quarter of human bones are in the feet.', true),
Question('A slug\'s blood is green.', true),
Question('Buzz Aldrin\'s mother\'s maiden name was \"Moon\".', true),
Question('It is illegal to pee in the Ocean in Portugal.', true),
Question(
'No piece of square dry paper can be folded in half more than 7 times.',
false),
Question(
'In London, UK, if you happen to die in the House of Parliament, you are technically entitled to a state funeral, because the building is considered too sacred a place.',
true),
Question(
'The loudest sound produced by any animal is 188 decibels. That animal is the African Elephant.',
false),
Question(
'The total surface area of two human lungs is approximately 70 square metres.',
true),
Question('Google was originally called \"Backrub\".', true),
Question(
'Chocolate affects a dog\'s heart and nervous system; a few ounces are enough to kill a small dog.',
true),
Question(
'In West Virginia, USA, if you accidentally hit an animal with your car, you are free to take it home to eat.',
true),
];
// 다음 질문 불러오기
void nextQuestion() {
if (_questionNumber < _questionBank.length - 1) {
// 질문 개수이내
_questionNumber++;
}
}
// _questionBank을 캡슐화 시켰고 변경운 불가능하게하고 불러만 오게끔 구현
String getQuestionText() {
return _questionBank[_questionNumber].questionText;
}
bool getQuestionNumber() {
return _questionBank[_questionNumber].questionAnswer;
}
}
import 'package:flutter/material.dart';
import 'package:quizzler/quiz_brain.dart';
QuizBrain quizBrain = QuizBrain();
void main() => runApp(Quizzler());
class Quizzler extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: Colors.grey.shade900,
body: SafeArea(
child: Padding(
padding: EdgeInsets.symmetric(horizontal: 10.0),
child: QuizPage(),
),
),
),
);
}
}
class QuizPage extends StatefulWidget {
@override
_QuizPageState createState() => _QuizPageState();
}
class _QuizPageState extends State<QuizPage> {
// 스코어 아이콘 리스트
List<Icon> scoreKeeper = [];
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Expanded(
flex: 5,
child: Padding(
padding: EdgeInsets.all(10.0),
child: Center(
child: Text(
quizBrain.getQuestionText(),
//리스트 이용
textAlign: TextAlign.center,
style: TextStyle(
fontSize: 25.0,
color: Colors.white,
),
),
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
textColor: Colors.white,
color: Colors.green,
child: Text(
'True',
style: TextStyle(
color: Colors.white,
fontSize: 20.0,
),
),
onPressed: () {
//The user picked true.
bool correctNumber = quizBrain.getQuestionNumber();
// if else 문을 활용한 정답 분기처리
if (correctNumber == true) {
print('right');
} else {
print('wrong');
}
setState(() {
quizBrain.nextQuestion();
});
},
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
color: Colors.red,
child: Text(
'False',
style: TextStyle(
fontSize: 20.0,
color: Colors.white,
),
),
onPressed: () {
//The user picked false.
setState(() {
quizBrain.nextQuestion();
});
},
),
),
),
// 스코어 리스트 동적으로 그려줌
Row(children: scoreKeeper)
],
);
}
}
/*
question1: 'You can lead a cow down stairs but not up stairs.', false,
question2: 'Approximately one quarter of human bones are in the feet.', true,
question3: 'A slug\'s blood is green.', true,
*/
[98. Inheritance in Action]
상속의 개념과 다트에서 어떻게 사용하는지에 대해 배웠습니다.
extends로 자바와 동일했습니다.
[99. Polymorphism in Action]
다형성에 대해 배웠습니다.
checkAnswer() 함수를 만들어 모듈화를 진행하였습니다.
import 'package:flutter/material.dart';
import 'package:quizzler/quiz_brain.dart';
QuizBrain quizBrain = QuizBrain();
void main() => runApp(Quizzler());
class Quizzler extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: Colors.grey.shade900,
body: SafeArea(
child: Padding(
padding: EdgeInsets.symmetric(horizontal: 10.0),
child: QuizPage(),
),
),
),
);
}
}
class QuizPage extends StatefulWidget {
@override
_QuizPageState createState() => _QuizPageState();
}
class _QuizPageState extends State<QuizPage> {
// 스코어 아이콘 리스트
List<Icon> scoreKeeper = [];
void checkAnswer(bool userPickedAnswer) {
//The user picked true.
bool correctAnswer = quizBrain.getQuestionNumber();
setState(() {
// if else 문을 활용한 정답 분기처리
if (userPickedAnswer == correctAnswer) {
scoreKeeper.add(Icon(
Icons.check,
color: Colors.green,
));
} else {
scoreKeeper.add(Icon(
Icons.close,
color: Colors.red,
));
}
// 다음 문제
quizBrain.nextQuestion();
});
}
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Expanded(
flex: 5,
child: Padding(
padding: EdgeInsets.all(10.0),
child: Center(
child: Text(
quizBrain.getQuestionText(),
//리스트 이용
textAlign: TextAlign.center,
style: TextStyle(
fontSize: 25.0,
color: Colors.white,
),
),
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
textColor: Colors.white,
color: Colors.green,
child: Text(
'True',
style: TextStyle(
color: Colors.white,
fontSize: 20.0,
),
),
onPressed: () {
//The user picked true.
checkAnswer(true);
},
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
color: Colors.red,
child: Text(
'False',
style: TextStyle(
fontSize: 20.0,
color: Colors.white,
),
),
onPressed: () {
//The user picked false.
checkAnswer(false);
},
),
),
),
// 스코어 리스트 동적으로 그려줌
Row(children: scoreKeeper)
],
);
}
}
/*
question1: 'You can lead a cow down stairs but not up stairs.', false,
question2: 'Approximately one quarter of human bones are in the feet.', true,
question3: 'A slug\'s blood is green.', true,
*/
[101. Code Challenge]
코드 챌린지로 알트 라이브러리를 추가하여 마지막 문제까지 풀면 알트창을 띄워주고 처음부터 다시 풀 수 있게 해주는 기능을 구현했습니다.
import 'question.dart';
class QuizBrain {
// 질문 번호 (핫리로드시 state를 보존하기때문에 이 값을 보존함 0부터 다시 시작하고싶으면 핫스타트 사용해야함)
int _questionNumber = 0;
// 캡슐화시킴
List<Question> _questionBank = [
Question('Some cats are actually allergic to humans', true),
Question('You can lead a cow down stairs but not up stairs.', false),
Question('Approximately one quarter of human bones are in the feet.', true),
Question('A slug\'s blood is green.', true),
Question('Buzz Aldrin\'s mother\'s maiden name was \"Moon\".', true),
Question('It is illegal to pee in the Ocean in Portugal.', true),
Question(
'No piece of square dry paper can be folded in half more than 7 times.',
false),
Question(
'In London, UK, if you happen to die in the House of Parliament, you are technically entitled to a state funeral, because the building is considered too sacred a place.',
true),
Question(
'The loudest sound produced by any animal is 188 decibels. That animal is the African Elephant.',
false),
Question(
'The total surface area of two human lungs is approximately 70 square metres.',
true),
Question('Google was originally called \"Backrub\".', true),
Question(
'Chocolate affects a dog\'s heart and nervous system; a few ounces are enough to kill a small dog.',
true),
Question(
'In West Virginia, USA, if you accidentally hit an animal with your car, you are free to take it home to eat.',
true),
];
// 다음 질문 불러오기
void nextQuestion() {
if (_questionNumber < _questionBank.length - 1) {
// 질문 개수이내
_questionNumber++;
}
}
// _questionBank을 캡슐화 시켰고 변경운 불가능하게하고 불러만 오게끔 구현
String getQuestionText() {
return _questionBank[_questionNumber].questionText;
}
bool getCorrectAnswer() {
return _questionBank[_questionNumber].questionAnswer;
}
//TODO: Step 3 Part A - Create a method called isFinished() here that checks to see if we have reached the last question. It should return (have an output) true if we've reached the last question and it should return false if we're not there yet.
bool isFinished() {
if (_questionNumber >= _questionBank.length - 1) {
//TODO: Step 3 Part B - Use a print statement to check that isFinished is returning true when you are indeed at the end of the quiz and when a restart should happen.
print('Now returning true');
return true;
} else {
return false;
}
}
//TODO: Step 4 part B - Create a reset() method here that sets the questionNumber back to 0.
void reset() {
_questionNumber = 0;
}
}
import 'package:flutter/material.dart';
//TODO: Step 2 - Import the rFlutter_Alert package here.
import 'package:rflutter_alert/rflutter_alert.dart';
import 'quiz_brain.dart';
QuizBrain quizBrain = QuizBrain();
void main() => runApp(Quizzler());
class Quizzler extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: Colors.grey.shade900,
body: SafeArea(
child: Padding(
padding: EdgeInsets.symmetric(horizontal: 10.0),
child: QuizPage(),
),
),
),
);
}
}
class QuizPage extends StatefulWidget {
@override
_QuizPageState createState() => _QuizPageState();
}
class _QuizPageState extends State<QuizPage> {
List<Icon> scoreKeeper = [];
void checkAnswer(bool userPickedAnswer) {
bool correctAnswer = quizBrain.getCorrectAnswer();
setState(() {
//TODO: Step 4 - Use IF/ELSE to check if we've reached the end of the quiz. If so,
//On the next line, you can also use if (quizBrain.isFinished()) {}, it does the same thing.
if (quizBrain.isFinished() == true) {
//TODO Step 4 Part A - show an alert using rFlutter_alert,
//This is the code for the basic alert from the docs for rFlutter Alert:
//Alert(context: context, title: "RFLUTTER", desc: "Flutter is awesome.").show();
//Modified for our purposes:
Alert(
context: context,
title: 'Finished!',
desc: 'You\'ve reached the end of the quiz.',
).show();
//TODO Step 4 Part C - reset the questionNumber,
quizBrain.reset();
//TODO Step 4 Part D - empty out the scoreKeeper.
scoreKeeper = [];
}
//TODO: Step 6 - If we've not reached the end, ELSE do the answer checking steps below 👇
else {
if (userPickedAnswer == correctAnswer) {
scoreKeeper.add(Icon(
Icons.check,
color: Colors.green,
));
} else {
scoreKeeper.add(Icon(
Icons.close,
color: Colors.red,
));
}
quizBrain.nextQuestion();
}
});
}
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Expanded(
flex: 5,
child: Padding(
padding: EdgeInsets.all(10.0),
child: Center(
child: Text(
quizBrain.getQuestionText(),
textAlign: TextAlign.center,
style: TextStyle(
fontSize: 25.0,
color: Colors.white,
),
),
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
textColor: Colors.white,
color: Colors.green,
child: Text(
'True',
style: TextStyle(
color: Colors.white,
fontSize: 20.0,
),
),
onPressed: () {
//The user picked true.
checkAnswer(true);
},
),
),
),
Expanded(
child: Padding(
padding: EdgeInsets.all(15.0),
child: FlatButton(
color: Colors.red,
child: Text(
'False',
style: TextStyle(
fontSize: 20.0,
color: Colors.white,
),
),
onPressed: () {
//The user picked false.
checkAnswer(false);
},
),
),
),
Row(
children: scoreKeeper,
)
],
);
}
}
/*
question1: 'You can lead a cow down stairs but not up stairs.', false,
question2: 'Approximately one quarter of human bones are in the feet.', true,
question3: 'A slug\'s blood is green.', true,
*/
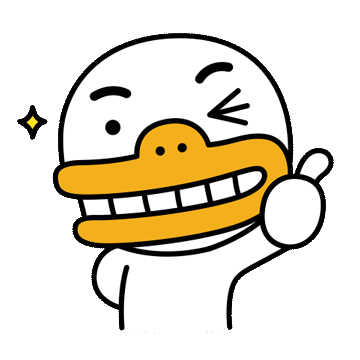
이상 섹션 10 강의를 학습완료했습니다.
댓글과 공감은 큰 힘이 됩니다. 감사합니다. !!!
[다음강의]
https://youngest-programming.tistory.com/632
[Flutter] Udemy 플러터 강의 섹션 11 학습 (학습중...) (섹션 11: Boss Level Challenge 2 - Destini)
[이전학습] https://youngest-programming.tistory.com/624 [Flutter] Udemy 플러터 강의 섹션 10 학습 (섹션 10: Quizzler -Modularising & Organising FlutterCode) [이전학습] https://youngest-programming.t..
youngest-programming.tistory.com